If you want to change the label and placeholder of the Dokan bank payment fields on the vendor setup wizard, you can do that using custom code.
You must insert the code on your child theme’s functions.php file.
/*
You can change any field title or remove any feild for the vendor -> settings -> payment -> bank transfer method. Please note that this
code need to be placed on your child-theme functions.php file
*/
/**
* Change the payment title
*
* @param array $methods
*
* @return array
*/
function dokan_2022_change_whithdraw_callback( $methods ) {
$methods ['bank']['title'] = __( 'Wire Transfer', 'dokan-lite' ); //title can be changed as per your need
return $methods;
}
add_filter( 'dokan_withdraw_methods', 'dokan_2022_change_whithdraw_callback', 12 );
/**
* Change the bank payment fields labels and placeholders
*
* @param array $fields
*
* @return array
*/
function dokan_2022_change_bank_payment_fields_placeholders_and_labels( $fields ) {
$fields['ac_name'] = [
'label' => __( 'Account Holder', 'dokan-lite' ),
'placeholder' => __( 'Your bank account name', 'dokan-lite' ),
];
$fields['ac_type'] = [
'label' => __( 'Account Type', 'dokan-lite' ),
'placeholder' => __( 'Account Type', 'dokan-lite' ),
];
$fields['ac_number'] = [
'label' => __( 'Account Number', 'dokan-lite' ),
'placeholder' => __( 'Account Number', 'dokan-lite' ),
];
$fields['routing_number'] = [
'label' => __( 'Routing Number', 'dokan-lite' ),
'placeholder' => __( 'Routing Number', 'dokan-lite' ),
];
$fields['bank_name'] = [
'label' => __( 'Bank Name', 'dokan-lite' ),
'placeholder' => __( 'Name of bank', 'dokan-lite' ),
];
$fields['bank_addr'] = [
'label' => __( 'Bank Address', 'dokan-lite' ),
'placeholder' => __( 'Address of your bank', 'dokan-lite' ),
];
$fields['iban'] = [
'label' => __( 'Bank IBAN', 'dokan-lite' ),
'placeholder' => __( 'IBAN', 'dokan-lite' ),
];
$fields['swift'] = [
'label' => __( 'Bank Swift Code', 'dokan-lite' ),
'placeholder' => __( 'Swift Code', 'dokan-lite' ),
];
$fields['declaration'] = [
'label' => __( 'I attest that I am the owner and have full authorization to this bank account', 'dokan-lite' ),
'placeholder' => __( '', 'dokan-lite' ),
];
$fields['form_caution'] = [
'label' => __( 'Please double-check your account information!', 'dokan-lite' ),
'placeholder' => __( 'Incorrect or mismatched account name and number can result in withdrawal delays and fees', 'dokan-lite' ),
];
return $fields;
}
add_filter( 'dokan_bank_payment_fields_placeholders', 'dokan_2022_change_bank_payment_fields_placeholders_and_labels' );
You can change the placeholder name by changing the “label” and “placeholders” in the code/
Here is the image of the setup wizard before adding the code-
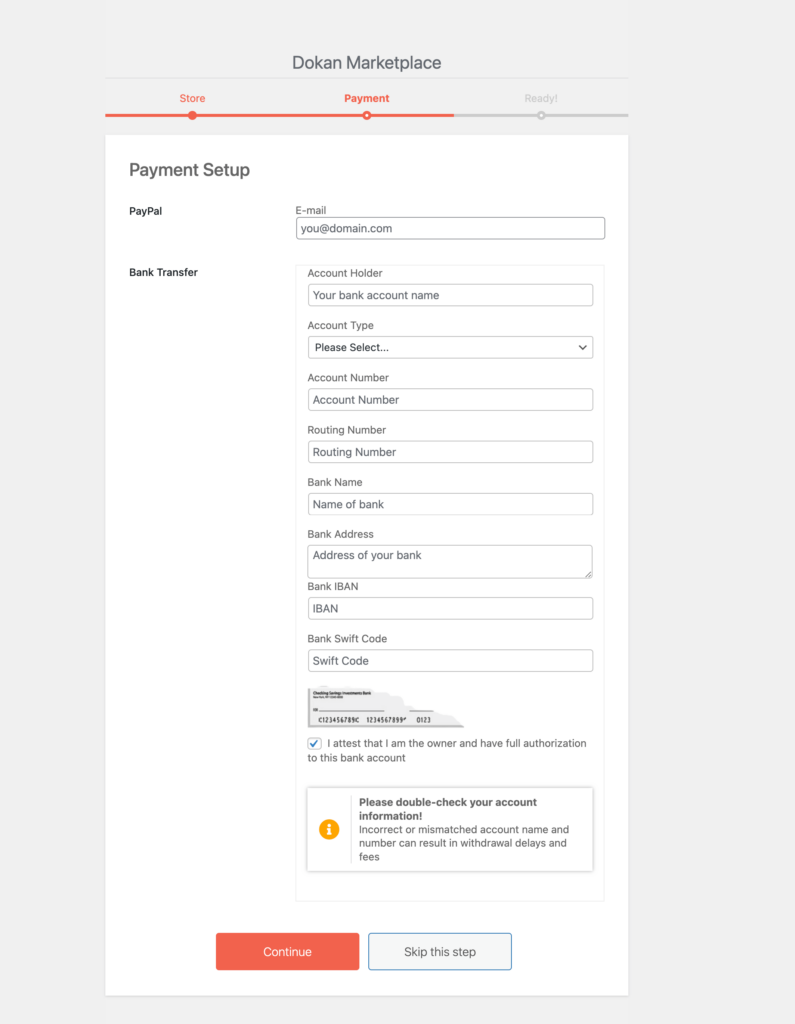
Here is the modified bank setup wizard-
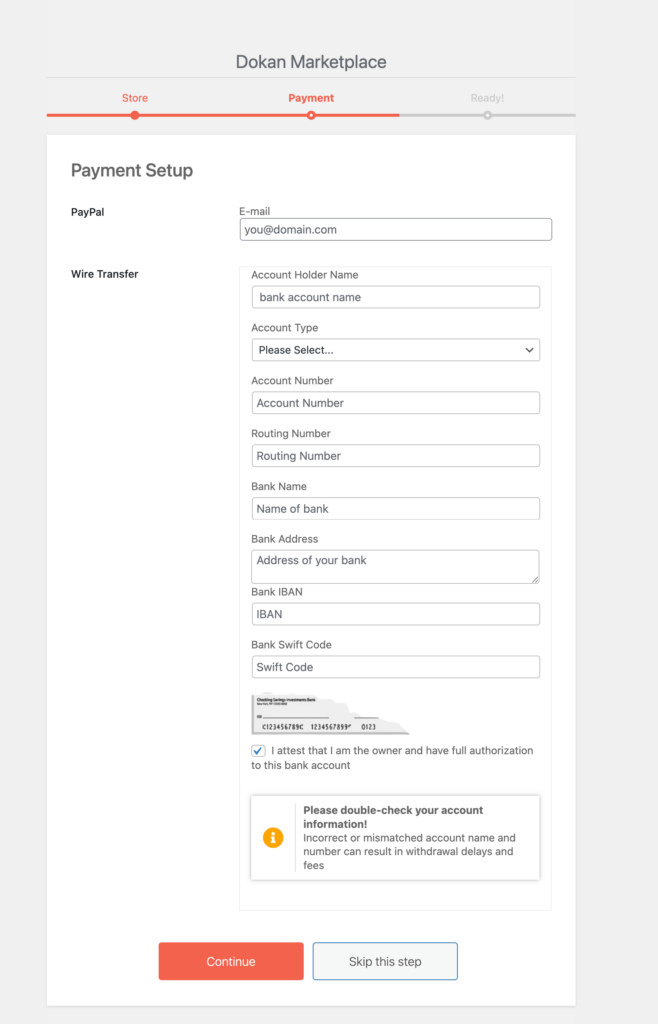
This is how to change the Label and Placeholder of Bank Payment Fields.
How to Make the Bank Payment Required Fields Unrequired
Use the below code to make any required fields in bank payment unrequired-
add_filter(
'dokan_bank_payment_required_fields', function ( $required_fields ) {
unset( $required_fields['ac_type'] );
return $required_fields;
}
);
Just replace the ‘ac_type’ with the field name you want to make it unrequited.
How to Make the Bank Payment Unrequired Fields Required
You can’t make changes like making bank/wire transfer required fields unrequired or vice-versa in the vendor setup wizard. But you can make changes in the vendor dashboard payment section.
Use the below code to make any unrequired fields in bank payment required-
add_filter(
'dokan_bank_payment_required_fields', function ( $required_fields ) {
$required_fields['iban'] = __( 'Iban is required', 'dokan-lite' );
return $required_fields;
}
);
Just replace the ‘iban’ with the field you want to make it required.